모든 데이터는 텐서로 표현할 수 있다.
넘파이리뷰
1D Array with NumPy
우선 넘파이를 임포트 해줘야 한다.
import numpy as np
import torch
print('Rank of t: ', t.ndim)
print('Shape of t: ', t.shape)
랭크가 의미하는것은 텐서의 차원을 의미하고 1차원으로 나열했기 때문에
랭크를 조회해보면 1로 결과가 나올것이다.
shape는 몇 개의 열이 있는가를 조회할 수 있다.
print('t[0] t[1] t[-1] = ', t[0], t[1], t[-1]) # Element
print('t[2:5] t[4:-1] = ', t[2:5], t[4:-1]) # Slicing
print('t[:2] t[3:] = ', t[:2], t[3:]) # Slicing
[]를 이용해서 슬라이싱을 할 수도 있다.
:뒤나 앞에 아무것도 입력하지 않으면 끝이나 처음을 의미한다.
결과 확인
2D Array with NumPy
t = np.array([[1., 2., 3.], [4., 5., 6.], [7., 8., 9.], [10., 11., 12.]])
print(t)
2차원으로 나열된 행렬의 랭크를 조회하면 2가 나올것이다.
shape를 이용하면 몇 행 몇 열인지 확인할 수 있다.
print('Rank of t: ', t.ndim)
print('Shape of t: ', t.shape)
결과 확인
파이토치를 이용해 표현해볼 수도 있다
PyTorch is like NumPy
1D Array with PyTorch
t = torch.FloatTensor([0., 1., 2., 3., 4., 5., 6.])
print(t)
print(t.dim()) # rank
print(t.shape) # shape
print(t.size()) # shape
print(t[0], t[1], t[-1]) # Element
print(t[2:5], t[4:-1]) # Slicing
print(t[:2], t[3:]) # Slicing
결과확인
t = torch.FloatTensor([[1., 2., 3.],
[4., 5., 6.],
[7., 8., 9.],
[10., 11., 12.]
])
print(t)
print(t.dim()) # rank
print(t.size()) # shape
print(t[:, 1])
print(t[:, 1].size())
print(t[:, :-1])
t = torch.FloatTensor([[[[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]],
[[13, 14, 15, 16],
[17, 18, 19, 20],
[21, 22, 23, 24]]
]])
print(t.dim()) # rank = 4
print(t.size()) # shape = (1, 2, 3, 4)
Frequently Used Operations in PyTorch
Mul vs. Matmul
그냥 mul은 단순한 곱셈을 의미하며
matmul은 행렬에서의 곱셈을 의미한다
연산자*를 사용하면 단산한 곱셈과 동일하다
print()
print('-------------')
print('Mul vs Matmul')
print('-------------')
m1 = torch.FloatTensor([[1, 2], [3, 4]])
m2 = torch.FloatTensor([[1], [2]])
print('Shape of Matrix 1: ', m1.shape) # 2 x 2
print('Shape of Matrix 2: ', m2.shape) # 2 x 1
print(m1.matmul(m2)) # 2 x 1
m1 = torch.FloatTensor([[1, 2], [3, 4]])
m2 = torch.FloatTensor([[1], [2]])
print('Shape of Matrix 1: ', m1.shape) # 2 x 2
print('Shape of Matrix 2: ', m2.shape) # 2 x 1
print(m1 * m2) # 2 x 2
print(m1.mul(m2))
Broadcasting
# Same shape
m1 = torch.FloatTensor([[3, 3]])
m2 = torch.FloatTensor([[2, 2]])
print(m1 + m2)
# Vector + scalar
m1 = torch.FloatTensor([[1, 2]])
m2 = torch.FloatTensor([3]) # 3 -> [[3, 3]]
print(m1 + m2)
# 2 x 1 Vector + 1 x 2 Vector
m1 = torch.FloatTensor([[1, 2]])
m2 = torch.FloatTensor([[3], [4]])
print(m1 + m2)
Broadcasting이란?
1. Broadcasting이란?
Broadcast의 사전적인 의미는 '퍼뜨리다'라는 뜻이 있는데, 이와 마찬가지로 두 행렬 A, B 중 크기가 작은 행렬을 크기가 큰 행렬과 모양(shape)이 맞게끔 늘려주는 것을 의미한다. 예를 들어, 아래의 행렬처럼 (3, 3)행렬에 (1, 3)행렬을 더하려고 할 때, (1, 3)행렬을 (3, 3)처럼 확장시켜 주는 것이 바로 브로드캐스팅(Broadcasting)이다. 따라서, 브로드캐스팅(Broadcasting)은 행렬의 덧셈 혹은 뺄셈에서 크기가 작은 행렬을 확장시켜 계산이 가능한 형태로 맞춰주는 것이다. 하지만, 모양(shape)을 확장하는 것은 가능하지만 축소하는 것은 데이터 손실 때문에 불가능하다.
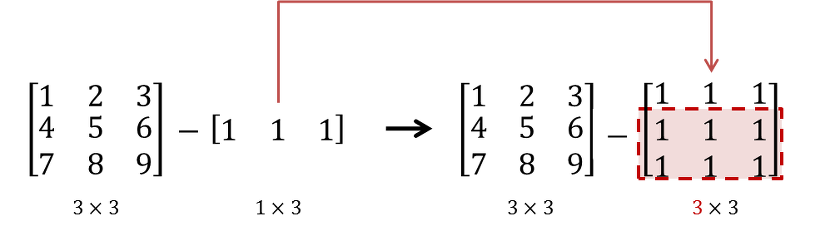
출처: https://excelsior-cjh.tistory.com/76 [EXCELSIOR]
Mean
mean은 말 그대로 평균을 의미한다.
t = torch.FloatTensor([1, 2])
print(t.mean())
tensor(1.5000)
# Can't use mean() on integers
t = torch.LongTensor([1, 2])
try:
print(t.mean())
except Exception as exc:
print(exc)
t = torch.FloatTensor([[1, 2], [3, 4]])
print(t)
print(t.mean())
print(t.mean(dim=0))
print(t.mean(dim=1))
print(t.mean(dim=-1))
차원을 설정해줌으로써 어떤 차원에서의 평균을 구할지 결정할 수 있다.
-1을 하게 되면 최고 차원으로 설정되는것을 확인할 수 있다.
코드
github.com/deeplearningzerotoall/PyTorch/blob/master/lab-01_tensor_manipulation.ipynb
deeplearningzerotoall/PyTorch
Deep Learning Zero to All - Pytorch. Contribute to deeplearningzerotoall/PyTorch development by creating an account on GitHub.
github.com
'ML > 모두의 딥러닝 시즌2' 카테고리의 다른 글
퍼셉트론(perceptron) (0) | 2021.01.22 |
---|---|
모두의 딥러닝 시즌2 [PyTorch] Lab-01-2 Tensor Manipulation 2 (0) | 2021.01.07 |
Docker Instruction (0) | 2021.01.05 |